oasting coffee is both an art and a science. The temperature and duration of the roasting process significantly impact the final flavor. But can we predict whether a coffee batch will have a good taste based on these two factors? In this blog, we'll build a simple neural network using TensorFlow to make this prediction.
We'll implement a feedforward neural network with:
Input Layer: Two features—roasting temperature and duration.
Hidden Layer: Three neurons with ReLU activation.
Output Layer: One neuron with a sigmoid activation to predict the probability of good coffee flavor.
This neural network uses forward propagation to compute its output. Let’s dive into the implementation!
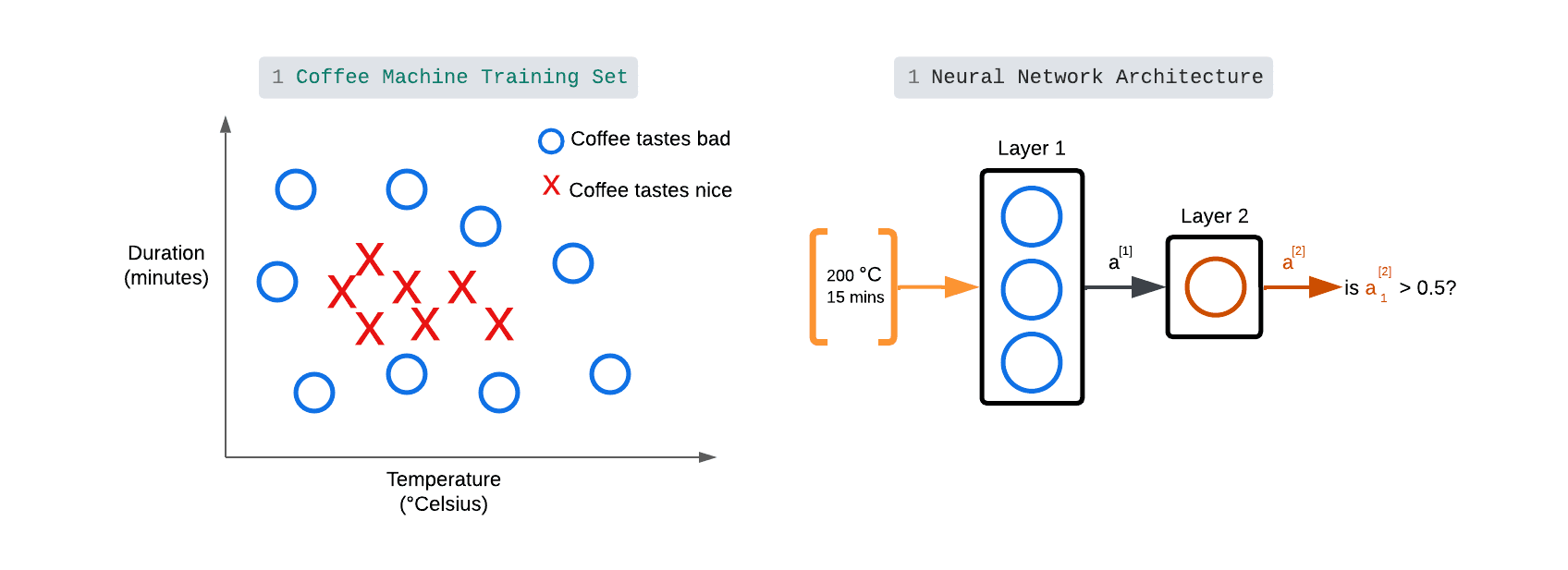
In the neural network architecture, X is the new pair of values we want the model to use to predict the new result
a1 is a vector with 3 numbers, each one the result of a calculation of a node in the first layer
a2 will be a scalar given that is the result of the output layer, which contains only one node that returns the final prediction.
Step 1: Setting Up Your Environment
Ensure you have TensorFlow installed. You can do this using pip install tensorflow.
Then import the libraries in your Python file.
import tensorflow as tf import numpy as np
Step 2: Define the Input Data and Labels
We’ll create a dataset where each sample consists of two inputs—roasting temperature and duration—and a label indicating whether the coffee had a good flavor (1) or not (0).
# Input features: temperature and grinding duration X = np.array([ [90, 15], [85, 12], [92, 10], [88, 20], [87, 18] ], dtype=np.float32) # Labels: 1 for good flavor, 0 otherwise y = np.array([ [1], [0], [1], [0], [0] ], dtype=np.float32)
A good way to think of this dataset is that if you cook the coffee at a low temperature, the beans won't get roasted, it ends up undercooked. If you cook it for not for long enough time, the duration is too short.
Finally, if you cook it either for too long time or at too high temperature, it will end up overcooked. Only points in the triangle will correspond to good coffee.
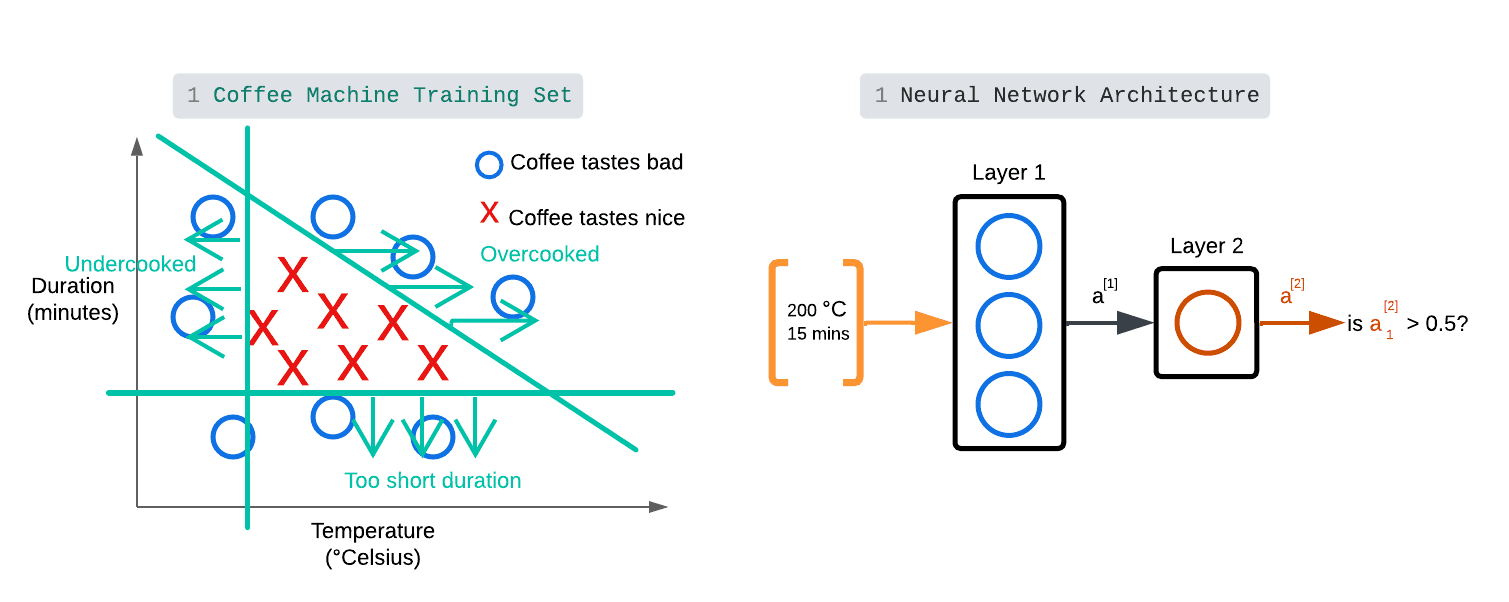
Step 4: Build the Neural Network
We’ll define a feedforward neural network with one hidden layer using TensorFlow’s Keras API.
First Layer: Dense layer with 3 nodes and ReLU activation.
Second Layer: Dense layer with 1 node and sigmoid activation for binary classification.
Dense is another name for layers in neural networks
# Define the model model = tf.keras.Sequential([ tf.keras.layers.Dense(3, activation='relu', input_shape=(2,)), # First layer tf.keras.layers.Dense(1, activation='sigmoid') # Second layer ]) layer_1 = Dense(units=3, activation='sigmoid')
Step 5: Compile the Model
We use the binary cross-entropy loss function since we’re dealing with binary classification. For optimization, we use Adam.
# Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
Step 6: Train the Model
Let’s train the model using forward propagation over 100 epochs.
# Train the model history = model.fit(X, y, epochs=100, verbose=1)
Step 7: Test the Model
Can the neural network learning algorithm optimize the quality of the beans you get from the roasting process? We can test the model with new inputs to predict whether the coffee will have a good flavor.
The task is, given a feature of vector X, with both temperature and duration, how can we do inference in a neural network to get it to tell us if this setting will result in good coffee or not?
# Test the model X = np.array([[89, 17], [93, 11]],) a1 = layer_1(X) a2 = layer_1(a1) predictions = model.predict(test_data) # Display predictions for i, pred in enumerate(predictions): print(f"Sample {i+1}: Probability of good flavor = {pred[0]:.2f}") if i >= 0.5: print("The data will result in good coffee") else: print("The settings will result in bad coffee")
How It Works
Input Data: The array
X
is fed into the model, where each row represents a sample with temperature and grinding duration.Hidden Layer: The first layer computes the weighted sum of inputs and applies the ReLU activation.
Output Layer: The second layer uses the sigmoid function to produce a probability score.
Prediction: Based on the sigmoid output, we can classify whether the coffee will have a good flavor.
Conclusion
This simple neural network gives us a starting point for predicting coffee flavor based on temperature and duration of the roasting process. Experiment with more features, adjust the architecture, or add regularization techniques to improve performance!